Ratings | | Unique User Downloads | | Download Rankings |
Not enough user ratings | | Total: 128 | | All time: 9,394 This week: 660 |
|
Description | | Author |
This package can register functions to call when conditions are met.
It provides a promise class that can register one callback function to be called when a condition is true. It can also call another callback function to be called when the condition is false.
The package also provides a deferred class to register a new promise to evaluate the conditions again later. Innovation Award
 August 2020
Number 3 |
A promise is a control structure that you can use in your code to define the actions to be executed when a condition is true, false, or needs to be evaluate later again while the condition status is not yet determined.
This package provides means to implement promises in PHP by evaluating conditions now or later using deferred promises.
Manuel Lemos |
| |
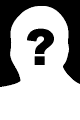 |
|
Innovation award
 Nominee: 9x |
|
Example
<?php
use SimplePromise\Deferred;
require 'vendor/autoload.php';
function test($number)
{
$deferred = new Deferred();
if ($number > 2){
$deferred->resolve('Succeeded');
}else{
$deferred->reject('Failed');
}
return $deferred->promise();
}
test(1)->then(function ($data){
echo "First: {$data} \n";
})->otherwise(function ($error){
echo "First: {$error} \n";
});
test(4)->then(function ($data){
echo "Second: {$data} \n";
})->otherwise(function ($error){
echo "Second: {$error} \n";
});
|
Details
Simple Promise
A simple PHP promise library that works synchronously.
Note
Please note that this library cannot be used in Asynchronous projects, projects like
ReactPHP or Amphp.
Installation
Make sure that you have composer installed
Composer.
If you don't have Composer run the below command
curl -sS https://getcomposer.org/installer | php
Run the installation
composer require ahmard/simple-promise ^1.0
Usage
<?php
use SimplePromise\Deferred;
require 'vendor/autoload.php';
function test($number)
{
$deferred = new Deferred();
if ($number > 2){
$deferred->resolve('Succeeded');
}else{
$deferred->reject('Failed');
}
return $deferred->promise();
}
test(1)->then(function ($data){
echo $data;
})->otherwise(function ($error){
echo $error;
});
|
Applications that use this package |
|
No pages of applications that use this class were specified.
If you know an application of this package, send a message to the author to add a link here.